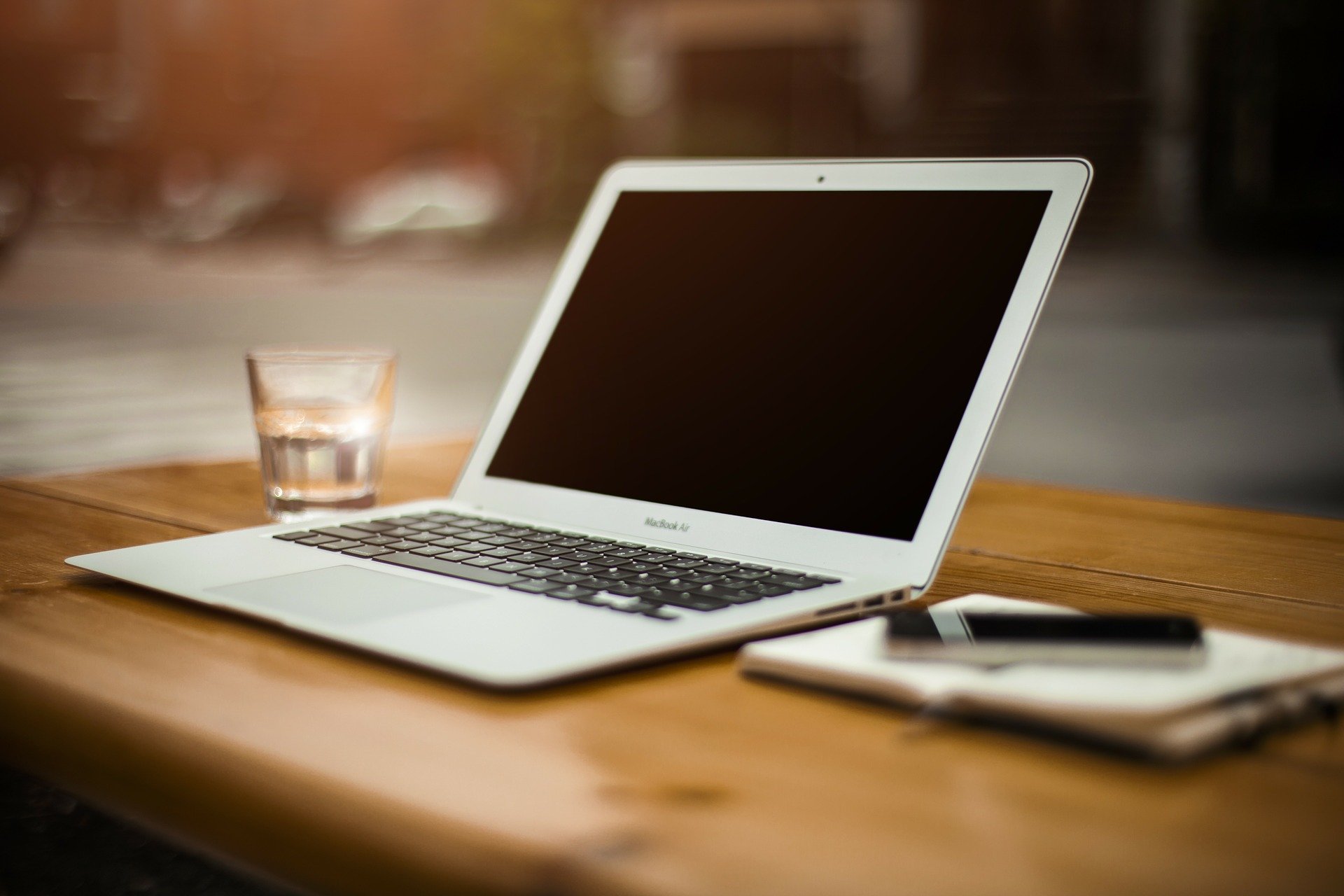
How to start a Django project
June 16, 2019, 10:02 a.m.
One thing is to read and understand (more or less) what you are doing / coding, and the other thing is to tell about it to another person or even to teach others.
As I wrote in my previous post (https://makneta.herokuapp.com/post/hello-world/) I’m going to write about creating and developing this blog that is written in Python3.7 and in Django2.2 framework on Ubuntu18.04.
And today is my first attempt.
There are only three simple steps to start a Django project:
1. Create virtual environment and activate it.
2. Install Django.
3. Start the project and create first application.
1. What is a virtual environment and why we should use it?
Despite standard library, Python has got many packages and modules that we can add and use in our projects. These libraries can have different version that we can use in different projects. I currently work on this blog and I use Django2.2 version and at the same time I have got another Django project where I use 1.11 version of Django. If I would install Django globally I wouldn’t been able to work on both of them at the same time because of version conflicts.
That’s why it is wiser to use a tool called Virtual Environment to separate each dependencies required by every project. Virtual Environment is like a drawer or a box where you put all stuff together to use them when needed instead of going through all the mess every time you want to work on your project.
There are different Virtual Environment packages to use, but I have chosen Pipenv.
Pipenv is more than Virtual Environment. It’s a dependency manager tool.
But before we start using Pipenv we have to check our Python version.
All code I’m presenting now is written in Command Line and the dollar sign $ is used as a prompt and if someone wants to copy my code shouldn’t copy this sign.
$ python --version
On my computer I have Python3.6 version but if you don’t please upgrade the version and then use pip or pip3 to install pipenv
$ pip install --user pipenv
Now create a folder where you are going to keep your django project and change into this project’s directory
$ mkdir mysite
$ cd mysite
If we want to use python3.7 in this project we should start from:
$ pipenv --python 3.7
And then activate the pipenv using:
$ pipenv shell
Now you should have the name of your folder in a parenthesis at the beginning of a line in command line.
(mysite) /mysite$
There should be also two files (packages) – Pipfile and Pipfile.lock. From this point whenever we use
$ pipenv install <package name>
it is automatically added to Pipfile and Pipfile.lock and we don’t need to have a requirements.txt file .
Why having these files is important?
If we want to work on the same project with another person (team) or we want to ask a more advanced developer for help and we add our code to Github (or other website with version control), those people won’t be able to make this project work for them without having all libraries with exact version installed on their machine.
More to read about Pipenv and Virtual Environment - https://docs.python-guide.org/dev/virtualenvs/
2. OK. Now we can install Django
If we want use the latest version of Django, it’s enough to write
$ pipenv install django
But if we want to use earlier version we should be more specified and for example write:
$ pipenv install "django==1.11"
or
$ pipenv install "django<3.0"
if want to use lastest version before 3.0.
3. And create our Django project
First we create project. This time we want to create another website so we want to call the project simply website. So we will write in terminal (command line)
$ django-admin startproject mywebsite .
The dot at the end of the line is there for purpose. It’s not a spot on a screen.
If we don’t use the dot we will create a folder called mysite with a subfolder called mysite with files essential for every Django project. So to avoid confusion we will add dot and we will create only one website folder with 4 files in it: __init__.py, settings.py, urls.py and wsgi.py. And at the same time the file manage.py is created.
From now on, if we want to do anything in our project from command line we have to be in the same folder with manage.py file.
We can open every file to read what is inside and find out that there are lots of things prepared and ready to use.
To check if everything was done correctly we can initialize our project using:
$ python manage.py runserver
We have information about unapplied migrations but we won’t do anything with this for the time being.
The most important information for us is written below:
And now we can open the browser and write the address: http://127.0.0.1:8000 or use open link command .
4. Creating Django app
Now when we successfully created our project we can create applications. It is said that every bigger functionality should be a new app. A blog should be one app and a newsletter or adding users should be another apps.
We start from creating Blog App writing in command line:
$ python manage.py startapp blog
Now we have another folder called blog with a few files in it. And there is also a new file called db.sqlite3. We can see that Django app has a built-in database. For learning purpose this database is enough, but later on we will change it to Postgresql database.
Now we can make first adjustment in our code. And today we’re going to make only a few changes in website/settings.py file.
Firstly, we can change allowed hosts in line 28 by adding our local host: '127.0.0.1'.
Secondly, we will add our blog app to installed apps (line 40)
We can also change language and timezone in our settings. If we change the language to our native tongue (assuming that our native language isn’t English) we will have all the admin stuff written in this language.
Next time I’m going to write about making a layout for our blog.
I hope I managed to write everything clearly. It would be really great if you let me know what do you think about this post.
PS. I'm publishing this post once again after I lost my posts because of migrations problems.
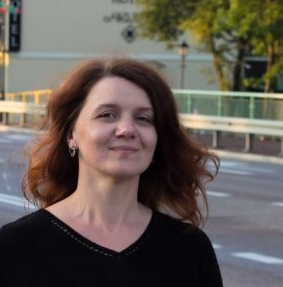
written by