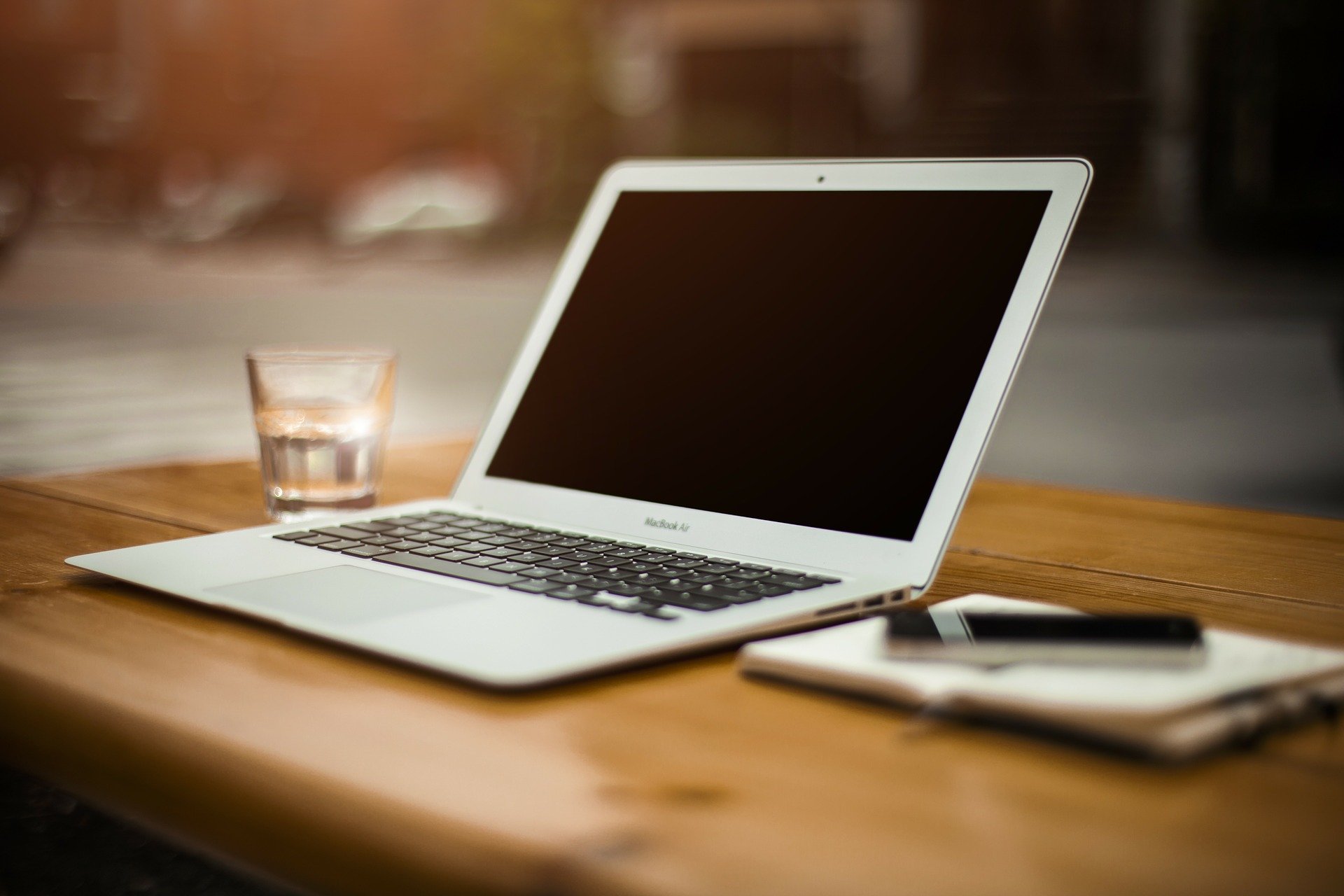
Unique string characters - kata in Python
May 19, 2020, 9:17 p.m.
The task:
There are two strings a and b; the task is to return the characters that are not common in the two strings.
The solution.
Let's start with talking a bit about strings.
A string is all characters stored between quotes. A single letter or number can be a string when it is taken in quotes. A word, a sentence or even a whole book can be a string. We can use double or single quotes around a string as long as we are consistent.
This is a string:
'word'
and this is a string:
"word"
But we can't write "word'. It will give us an error.
What are the features of a string:
* strings are immutable. It means we cannot modify them. If we change something in a string we create a new one;
* strings consist of individual characters, and we can access them using slicing. We must remember that the access range starts from zero;
* strings are iterable, so we can use for loops on them to check each character of the string;
* we can use + and * operators on strings. The + operator combines two strings into one, and it is called concatenation. The * operator repeats the string a certain number of times. It looks like this: print("Python" * 2 ) -- output PythonPython
* we can use the in operator to check if a string contains something.
I will use three last features to solve the puzzle.
In our task, we have given two strings. Those strings are passed into our function as parameters.
def solve(a, b):
def - it's the way to start function in Python;
solve - it's the name of the function. We can use the name we want, but it should be self-explicit, and it should be written lowercase;
(): - there should be parentheses and colon. Some functions can be without parameters, but in our function, we have two parameters a and b.
a and b are variables that contain strings, and we can glue them together. We can create a new variable:
ab = a + b
We have to find letters (characters) that are present only in string a or in string b.
Now we can iterate through ab variable and check if a character is not in a or not in b string.
The parenthesises are optional. I'm using them only for readability.
Now we need to store the unique characters somehow. So before for loop, we will create a new variable. I'll call it new, and the variable will equal an empty string:
new = ""
So far our function looks like this:
When we check if a character (letter) is only in one of the given strings, we have to add this letter to a variable called new.
We could write
new = new + char
but we can make it shorter and write like this:
new += char
And our function will return a new string.
def solve(a, b):
Another way of doing it (but very similar) is assigning an empty list to a variable called new.
We can skip creating ab variable remembering that + operator will concatenate both strings.
Now we cannot simply add a new character to the list. We have to use .append() method:
new.append(char)
But we are not asked about returning a list of letters. So now we again have to glue all list elements together. And we will use .join() method.
In this method, first, we have to decide what separator we are going to use. In our case, the separator will be an empty string. And then in parentheses, we write the name of a list we want to join.
return "".join(new)
The whole function:
The effect is the same. And there is almost the same amount of lines of code. Now we can refactor our code and create a one-liner.
How do we creat one-liner?
When we want to iterate through elements in a string or a list, we can use one-liner. It will create a new list.
If we want to make a list from a "Python" string we can use a for loop like this:
The same we can achieve in such a way:
It eliminates the usage of the append method.
We can also check if statements in a one-liner. We can, for example, decide that we don't want vowels.
With for loop:
One liner:
Now we can change our list back into a string using .join() method. This time we will put all our one-liner inside the parenthesis
And we can do the same with our Unique Characters task:
Working code is available:
https://repl.it/@maknetaRo1/FarflungKindCharacter
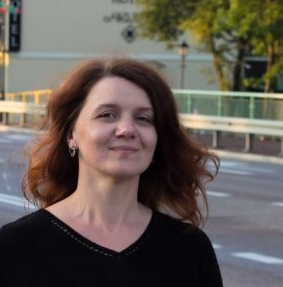
written by